XPath is a strong tool in itself, but you can even use
variables in your XPath expressions. The way it works is by referring to a variable as:
You use the variable element to assign a value to a variable. Variables are case-sensitive, so e.g. $var is not the same as $Var.
When assigning a variable value, you need to type in a valid XPath expression. For example, you can assign a numeric value to a variable without any delimiters. Still, if you want to assign an alphanumeric value, such as a string, you need to use delimiters around the string. You can use either ' or " to delimit the string.
Example of Assigning a Variable with an XPath Function
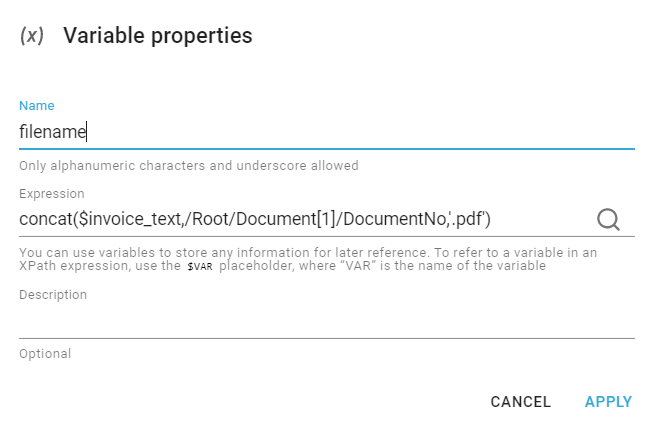
Here, the value of the variable filename is set as the value of the variable invoice_text (which in this case is language-dependent and contains, for example, the value "Invoice" or "Rechnung"), concatenated with the document number found in the XML file, and finally, the extension .pdf is added. This means a possible value of filename could be Invoice1001.pdf or Rechnung1001.pdf.
The example above is based on the demo XML file, which has multiple Document nodes, each with a DocumentNo. Therefore, this expression is not valid:
concat($invoice_text, /Root/Document/DocumentNo, '.pdf')
The reference /Root/Document/DocumentNo actually returns a list of four values.
To select only one value, use the following:
concat($invoice_text, /Root/Document[1]/DocumentNo, '.pdf')
With Document[1], we refer to the first occurrence of the Document node.
Handling Quotes in XML Nodes
If a node in your input XML file contains text with both single and double quotes, you need to replace these values with two other characters that will never appear in the node. For example, consider this text inside a node:
"Mary's lam had a littl' lam" (including the double quotes).
To safely insert this into a variable and later use it in XPath expressions, one way to handle this is:
var1 = translate(/Root/Node, '"', '#')
With this XPath expression, the sentence is converted into:
#Mary's lam had a littl' lam#
Now, apply a second translation:
var1 = translate($var1, "'", '£')
With this last translation, any single quote is converted into £:
#Mary£s lam had a littl£ lam#
Now you can translate it back when using var1 in an expression like this:
concat($Start, ' ', translate(translate($test, '#', '"'), "£", "'"))
If the variable Start
contained This is the sentence:
, then the result would be:
This is the sentence: "Mary's lam had a littl' lam"
Alternative Approach
Another simple way is to output the texts in a flow area without the need to use concat.